HIT-OSLab8
实验目的:
- 掌握虚拟文件系统的实现原理
- 实践文件、目录、文件系统等概念
实验内容:
- 在 Linux-0.11 上实现 procfs(proc文件系统) 内的 psinfo 节点,当读取此节点的内容的时候,可得到系统当前所有进程的状态信息
- 在 Linux-0.11 上实现 procfs(proc文件系统) 内的 hdinfo 节点,当读取此节点的内容的时候,可以打印出硬盘的一些信息
- 参考格式如下:
1 | cat /proc/psinfo |
实验过程
procfs 是一个 Linux 内核模块,它提供了一个用于访问进程信息的文件系统
- 通过 procfs,你可以查看与进程有关的信息,如进程 ID、进程名、进程的命令行参数等
- 它使得用户可以方便地对进程进行管理和监控
procfs 的实现依赖于内核的 proc 文件系统,它将进程信息存储在 /proc
目录下
- 这个目录提供了一组用于访问进程信息的文件和目录
- 通过这些文件,你可以查看进程的详细信息,如
/proc/[pid]/cmdline
文件可以查看进程的命令行参数
在开始实验前,我们需要先了解 linux 中的两个关键结构 file 和 inode
在 Linux 中,结构体 file 用于描述一个内存中的文件(打开的文件)
1 | struct file { |
每种类型的文件都有一个唯一的标识符,即索引节点 inode,结构体 inode 用于描述一个在磁盘中的文件或者一个有特定功能的虚拟文件
索引节点有两种类型:
- metadata inode 是用于存储文件元数据的 inode
- 元数据(metadata):用来描述一个文件的特征,包含了该文件或目录的元数据,如权限、所有者、组、大小、创建时间等
- data inode 是用于存储文件数据的 inode
- 数据(data):泛指普通文件中的实际数据
在 linux-0.11 中似乎没有刻意去区别这两种类型的 inode:
1 | struct d_inode { |
首先 proc 文件系统中包含一种特殊类型的文件,需要在 include/sys/stat.h
中进行定义:
1 |
接下来我们需要在 sys_mknod sys_read
中添加有关 proc 文件的判断,使其可以支持 proc 文件:
1 | int sys_mknod(const char * filename, int mode, int dev) |
1 | int sys_read(unsigned int fd,char * buf,int count) |
然后我们需要在根文件系统加载后创建 /proc
目录以及其中的 psinfo hdinfo
文件:
1 | setup((void *) &drive_info); /* 挂载根文件系统 */ |
最后的实验操作就是实现 proc_read
函数(新建 fs/proc.c
文件):
1 |
|
最后修改 fs/makefile
:
1 | OBJS= open.o read_write.o inode.o file_table.o buffer.o super.o \ |
效果如下:
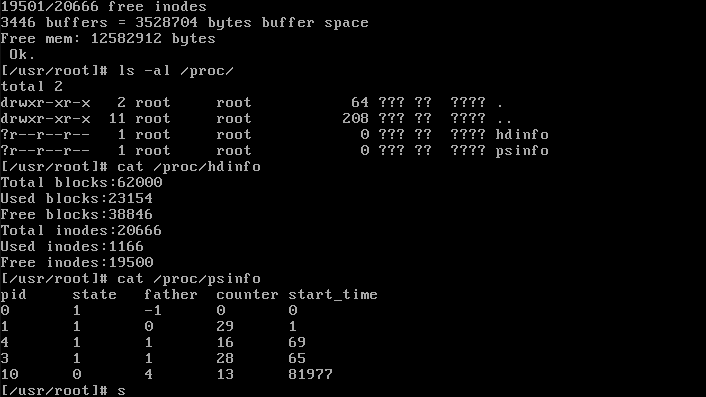