HIT-OSLab7
实验目的:
- 加深对操作系统设备管理基本原理的认识,了解键盘中断、扫描码等概念
- 通过实践掌握 Linux-0.11 对键盘终端和显示器终端的处理过程
实验内容:
- 本实验的基本内容是修改 Linux-0.11 的终端设备处理代码,对键盘输入和字符显示进行非常规的控制
- 在初始状态,一切如常,用户按一次 F12 后,把应用程序向终端输出所有字母都替换为
*
- 再按一次 F12,又恢复正常
- 第三次按 F12,再进行输出替换,依此类推
实验过程
终端设备是计算机系统中与用户交互的硬件设备,通常使用键盘和显示器来接收用户的输入和输出操作
在 Linux 系统中,每个终端设备都对应一个 tty_struct
结构体实例,用于存储该终端设备的状态、配置信息以及与终端相关的数据结构
1 | struct tty_struct |
1 | struct tty_queue |
扫描码是指计算机在接收用户的输入时,将用户的输入转换为计算机能够理解的形式,在键盘上,每个键都对应一个唯一的扫描码,这个扫描码可以用来识别用户按下的是哪个键
在操作系统中,键盘中断通常是通过中断处理程序(Interrupt Handler)来实现的
- 当用户按下键盘上的键时,计算机系统会向操作系统发送一个中断信号,操作系统接收到这个信号后,会调用相应的中断处理程序来处理这个中断事件
下面是键盘中断的处理程序代码:
1 | keyboard_interrupt: |
其中 key_table
存储有各个扫描码的处理程序:
1 | key_table: |
- 我们可以发现 F12 会调用
func
函数
核心思路就是添加一个 F12 标记位,我们可以选择将 func
替换为我们需要的函数,其目的是修改该标记位以记录用户是否输入了 F12
1 | int f12_key = 0; |
1 | .long change_f12_key,none,none,none /* 58-5B f12 ? ? ? */ |
函数 con_write
用于将字符串写入终端设备:
1 | void con_write(struct tty_struct * tty) |
- 其
case 0
用于处理 asicc 在(31,127)
范围的字符 - 我们需要在这段代码前检查
f12_key
并将字符设置为*
1 | void con_write(struct tty_struct * tty) |
最终效果如下:
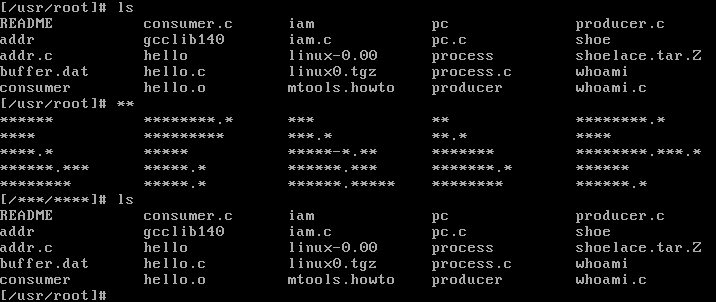